Making Sensor Lights in Unity 3D
Lighting in Unity 3D can some times get tricky. I just spent hours into making sensor lights only to come up with a very obvious solution.
At first, I was using baked maps which were the primary reason for my trouble.
I know it's kind of obvious but I need to state it for anyone who is struggling with the same.
Don't use Baked Lightmaps if you want real-time illumination.
As simple as that. I even considered lighting up the environment by real-time switching different lightmaps. Thanks to the complexity of such task I didn't do it.
Finally, the solution was to use simple point lights. To give the lights better look I used emissive materials.
Here are the results.
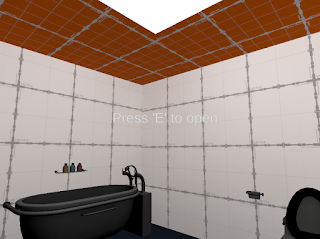
Here's how I did it.
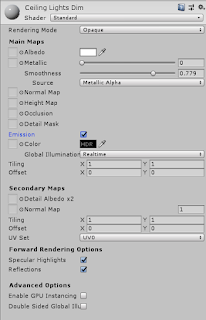
In case my naming of objects and variables is causing any confusion, there is two sources of light in this, cuboid and a point light. Originally I wanted to just light up the room using cuboid with emissive material but that doesn't work in real time lighting as they need to be baked. So I ended up using a point light to illuminate the room in real time and cuboid with emissive material to make it look better.
At first, I was using baked maps which were the primary reason for my trouble.
I know it's kind of obvious but I need to state it for anyone who is struggling with the same.
Don't use Baked Lightmaps if you want real-time illumination.
As simple as that. I even considered lighting up the environment by real-time switching different lightmaps. Thanks to the complexity of such task I didn't do it.
Finally, the solution was to use simple point lights. To give the lights better look I used emissive materials.
Here are the results.
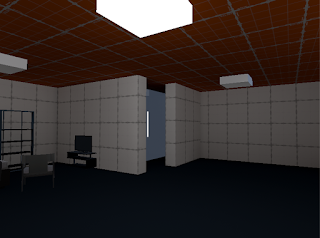
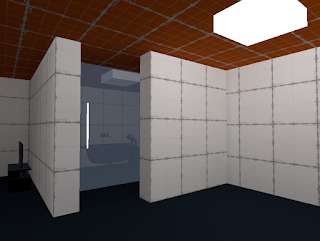
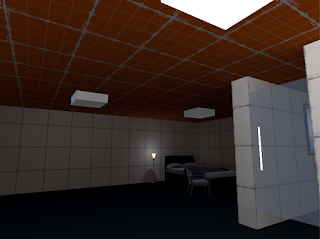
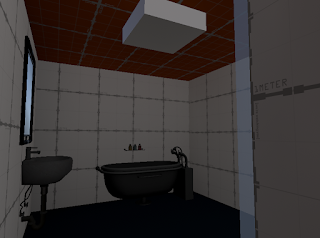
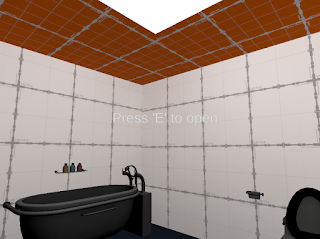
Here's how I did it.
- Created an empty Gameobject Light Controller and under that created two small cuboid, LightsOn and LightsOff and a point light called Bulb here.
- Added a box collider in Light Controller and ticked Is Trigger.
-
Here's the script attached to the Light Controller.
public class LightsOn : MonoBehaviour
{
public GameObject lightOn, lightOff, light;
private bool lightsOn = false;
private void OnTriggerEnter(Collider other)
{
if(other.tag == "Player" || lightsOn == false)
{
lightOn.SetActive(true);
lightOff.SetActive(false);
light.SetActive(true);
}
}
private void OnTriggerExit(Collider other)
{
if (other.tag == "Player" || lightsOn == true)
{
lightOff.SetActive(true);
lightOn.SetActive(false);
light.SetActive(false);
}
}
}
What this script does is, take checks if the Player enters the trigger collider and enables lightsOn object and bulb(light) and disables lightsOf object and bulb(light) and vice versa.
I also created two emissive materials with the following the property. They're just new material with albedo set to white and HDR Color set to white in lit up light(cuboid object above) and Black in dim light.
I also created two emissive materials with the following the property. They're just new material with albedo set to white and HDR Color set to white in lit up light(cuboid object above) and Black in dim light.
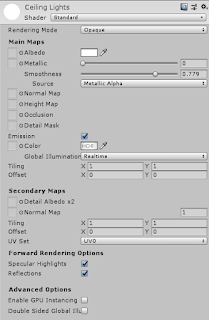
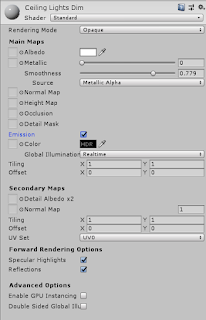
In case my naming of objects and variables is causing any confusion, there is two sources of light in this, cuboid and a point light. Originally I wanted to just light up the room using cuboid with emissive material but that doesn't work in real time lighting as they need to be baked. So I ended up using a point light to illuminate the room in real time and cuboid with emissive material to make it look better.
Hope this helps anyone looking into sensor lights. In case of any query feel free to comment below.